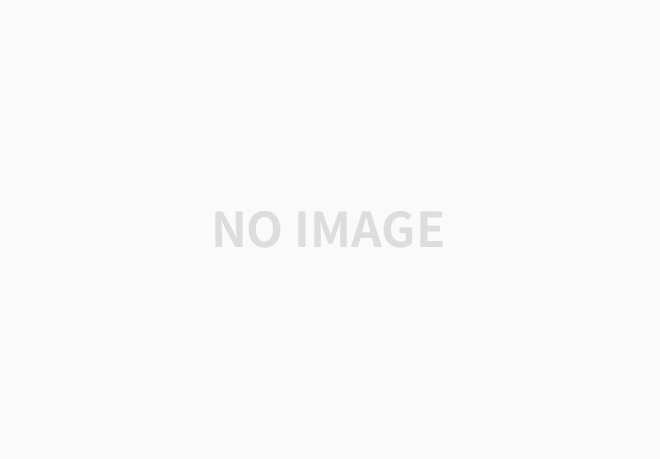
배열(Array)
- 하나의 이름으로 동일한 자료형의 데이터를 여러 개 연속적으로 저장할 수 있는 메모리 공간을 할당 받는 것을 말함
- 변수는 하나의 값만을 저장하지만, 배열은 여러 개의 값을 저장할 수 있음
- 같은 자료형의 데이터들이 순차적으로 저장됨
- 배열은 선언과 동시에 저장할 수 있는 데이터 타입이 결정이 됨
만약 다른 타입의 데이터를 저장하려면 타입 불일치 컴파일 오류가 발생함
- 배열의 방의 이름은 0이라는 인덱스부터 시작함
- 배열의 단점 : 배열은 한 번 크기가 정해지면 크기를 늘리거나 줄일 수 없음
배열의 선언과 생성
- 1단계 : 배열 선언(자료형[] 배열명 or 자료형 배열명[])
선언방법 | 선언 예 |
타입[] 변수이름; | int [] score; String[] name; |
타입 변수이름[]; | int score[]; String name[]; |
- 2단계 : 배열 메모리 생성(메모리 할당 : 배열명 = new 자료형[배열의 크기])
타입[] 변수이름; // 배열 선언(배열을 다루기 위한 참조변수 선언)
변수이름 = new 타입[]; // 배열 메모리 생성(실제 저장공간을 생성)
보통 1+2단계를 합쳐 한 줄로 간단하게-
타입[] 변수이름 = new 타입[길이];
로 배열의 선언과 생성을 한다.
- 3단계 : 배열 초기화(할당된 메모리 영역에 데이터를 저장)
- 4단계 : 배열을 이용(데이터 처리 = 연산, 출력 등)
배열의 선언과 생성 예시)
public static void main(String[] args) {
//1단계. 배열 선언(형식: 자료형[] 배열명(배열이름), 자료형 배열명[])
//int[] score;
//2단계. 배열 메모리 생성(메뫼 할당 : 배열명=new 자료형[배열의 킈기])
//score=new int[5];
//1단계 + 2단계 : 배열 선언 및 배열 메모리 생성
int[] score=new int[5];
System.out.println("배열 주소 >>>" + score); //배열주소
//3단계. 배열 초기화(할당된 메모리 영역에 데이터를 저장)
score[0]=10;
score[1]=20;
score[2]=30;
score[3]=40;
score[4]=50;
//4단계. 배열을 이용(데이터 처리-연산, 출력 등등)
score[2]+=100; //score[2]=score[2]+100
score[3]+=50; //score[3]=score[3]+50
System.out.println("score[0]>>>" +score[0]);
System.out.println("score[1]>>>" +score[1]);
System.out.println("score[2]>>>" +score[2]);
System.out.println("score[3]>>>" +score[3]);
System.out.println("score[4]>>>" +score[4]);
System.out.println();
//반복문을 이용하여 배열을 출력해보자.
for(int i=0; i<=4; i++) {
System.out.printf("score[%d] >>> %d\n", i, (score[i]));
}
}
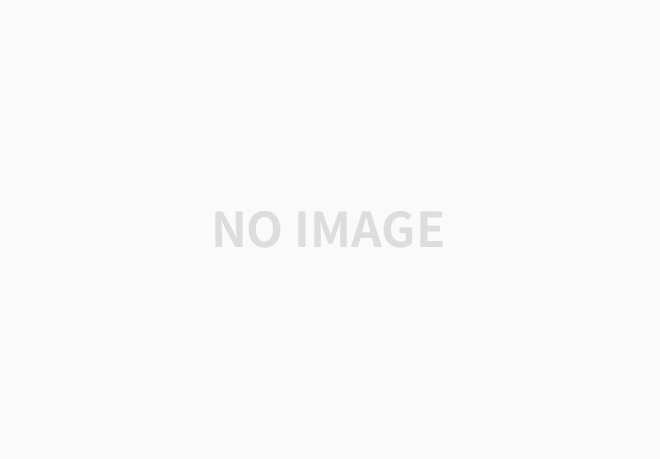
제일 첫 줄은 배열의 주소를 의미 ('타입@주소' 형식으로 출력됨)
I는 1차원 int배열이라는 의미이고,
@ 뒤에 나오는 16진수는 배열의 주소인데, 실제 주소가 아닌 내부 주소
배열의 생성 방법은 두가지 방법이 있는데,
1. new 키워드를 사용하여 배열 생성 - 위 방법
2. 배열의 초기값을 이용하여 배열 생성
ex)
public static void main(String[] args) { //배열을 생성함과 동시에 초기값 설정 int[] arr= {10, 20, 30, 40, 50}; arr[4]+=50; //arr[4]=100 으로 재지정 for(int k:arr) { //단축 for문 System.out.println("arr 배열의 값 : " + k); } }
배열의 초기화
int[] arr = new int[] {10, 20, 30, 40, 50} ; //배열의 생성과 초기화를 동시에
{} 괄호 안의 값의 개수에 의해 배열의 길이가 자동으로 결정되기 때문에
[] 괄호 안의 배열의 길이는 안 적어도 된다.
int[] arr = {10, 20, 30, 40, 50} ; // new int[]를 생략할 수 있음
배열의 길이
* length 속성
- 배열의 크기(길이)를 알려주는 키워드.
- 배열의 크기(길이)를 정수값으로 알려줌.
- 이미 생성된 배열의 길이는 변하지 않기 때문에, '배열이름.length'는 상수값.
즉, 값을 읽을 수만 있을 뿐 변경할 수는 없음
int arr = new int[5];
arr.length = 10; //에러. 배열의 길이는 변경할 수 없음
형식) 배열명.length
예) int size = arr.length
public static void main(String[] args) {
int[] arr=new int[3];
System.out.println("arr 배열의 크기(길이) >>> "+arr.length);
}
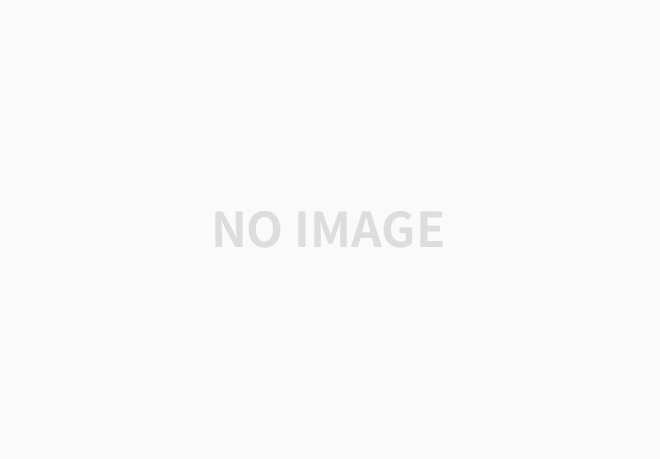
* 배열의 길이 변경하기
- 한번 선언된 배열은 길이를 변경할 수 없음
- 만일 변경하고 싶을 경우, 새로운 배열을 생성한 다음
기존의 배열에 저장된 값들을 새로운 배열에 복사하면 됨
예제01)
키보드로 배열의 크기와 값 입력받기
import java.util.Scanner;
// 키보드로 배열의 크기를 입력받아 보자
public class Ex06 {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
System.out.print("배열의 크기를 입력하세요. >>> ");
int[] arr=new int[sc.nextInt()];
System.out.println("arr 배열의 크기(길이) >>> "+arr.length);
System.out.println();
for(int i=0; i<arr.length; i++) {
System.out.print((i+1)+"번쨰 정수 입력>>> ");
arr[i]=sc.nextInt();
}
System.out.println();
for(int i=0; i<arr.length;i++) {
System.out.printf("arr[%d] >>> %d\n",i,arr[i]);
}
sc.close();
}
}
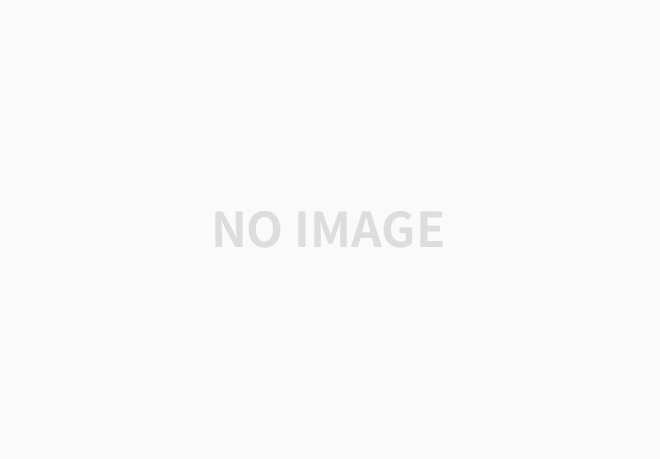
예제 02)
배열에 저장된 데이터 화면에 출력해보기
import java.util.Scanner;
public class Ex07 {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
System.out.print("문자열 배열의 크기를 입력하세요. : ");
String[] str=new String[sc.nextInt()];
System.out.println();
//문자열 배열에 데이터를 저장해 보자
for(int i=0; i<str.length; i++) {
System.out.printf("%d번째로 입력할 문자열을 입력해주세요. ", i+1);
str[i]=sc.next();
}
System.out.println();
//문자열 배열에 저장된 데이터를 화면에 출력해보자.
for(int i=0; i<str.length; i++) {
System.out.printf("str[%d] >>> %s\n", i, str[i]);
}
System.out.println();
//문자열 배열에 저장한 문자열을 검색을 해 보자.
System.out.print("검색할 문자열을 입력하세요. ");
String search=sc.next();
String result="default";
for(int i=0; i<str.length; i++) {
if(search.equals(str[i])) {
result="true";
System.out.printf("%s(은)는 %d번째로 입력하셨습니다.",search,(i+1));
// 배열 인덱스는 0부터 시작하므로 i+1로 입력
}
}//for문 종료
if(result=="defualt") { //못 찾았을 시 실행문
System.out.printf("입력된 데이터 %s(은)는 배열에 없습니다.\n",search);
}
sc.close();
}
}
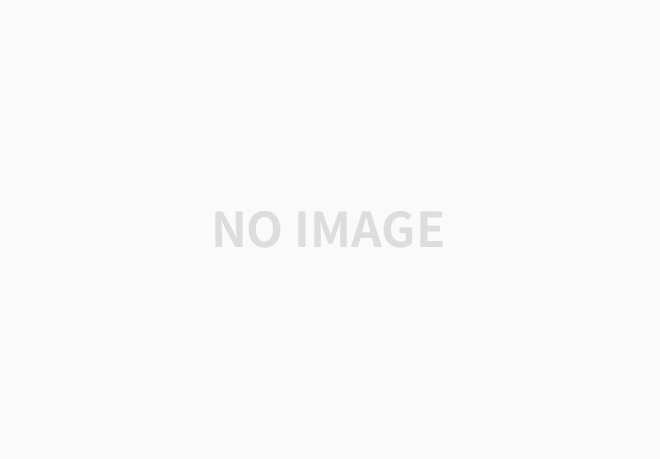
'Back-End > Java' 카테고리의 다른 글
[Java] 배열 공유 (0) | 2021.08.16 |
---|---|
[Java] 단축 for문(개선된 for문, for each문) (0) | 2021.08.11 |
[Java] 기타보조제어문(continue, break) (0) | 2021.08.11 |
[Java] 조건문 for문 (0) | 2021.08.08 |
[Java] 반복문 while, do while (0) | 2021.08.08 |
댓글